

Common cathode
Common anode
H-Segment dot led is Enable Table
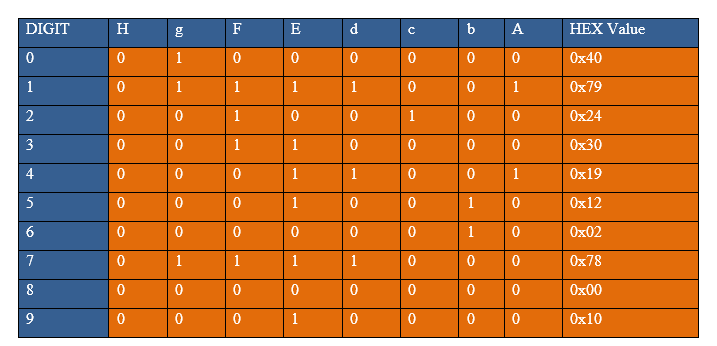
H-Segment dot led is Disable Table

/* Name : main.c * Purpose : Source code for 7SEG-COMMON-ANODE Interfacing with PIC18F4550. * Author : Gemicates * Date : 2017-06-09 * Website : www.gemicates.org * Revision : None */ #include <htc.h> // Header file for PIC18F4550 #define _XTAL_FREQ 12000000 // 12MHZ #define seg_port PORTD // To assign seg_port as PORTD //__CONFIG(PLLDIV = 5,CPUDIV = OSC1 / 2,USBDIV = 2,FOSC = HIGH_SPEED HS); //,FCMEN = OFF,IESO = OFF,PWRT = OFF,BOR = OFF,BORV = 3,VREGEN = OFF,WDT = OFF,WDTPS = 1:32768,CCP2MX = ON,PBADEN = OFF,LPT1OSC = OFF,MCLRE = OFF,STVREN = ON,LVP = OFF,ICPRT = OFF,XINST = OFF,DEBUG = OFF,CP0 = OFF, CP1 = OFF, CP2 = OFF, CP3 = OFF,CPB = OFF,CPD = OFF,WRT0 = OFF, WRT1 = OFF, WRT2 = OFF, WRT3 = OFF,WRTC = OFF,WRTB = OFF,WRTD = OFF,EBTR0 = OFF, EBTR1 = OFF, EBTR2 = OFF, EBTR3 = OFF,EBTRB = OFF); #pragma config WDT = OFF void delay(int msec) // delay function declaration { int i,j; for(i=0;i<msec;i++) for(j=0;j<1275;j++); } void main() { TRISD = 0x00; // PORTD as output seg_port = 0xff; // PORTD as input while(1) { seg_port = 0xFE; // 'A' segment turn on delay(80); seg_port = 0xFD; // 'B' segment turn on delay(80); seg_port = 0xFB; // 'C' segment turn on delay(80); seg_port = 0xF7; // 'D' segment turn on delay(80); seg_port = 0xEF; // 'E' segment turn on delay(80); seg_port = 0xDF; // 'F' segment turn on delay(80); seg_port = 0xBF; // 'G' segment turn on delay(80); seg_port = 0x7F; // 'H' segment turn on delay(100); // Default 'H' segment turn OFF seg_port = 0x40; // Display '0' delay(80); seg_port = 0x79; // Display '1' delay(80); seg_port = 0x24; // Display '2' delay(80); seg_port = 0x30; // Display '3' delay(80); seg_port = 0x19; // Display '4' delay(80); seg_port = 0x12; // Display '5' delay(80); seg_port = 0x02; // Display '6' delay(80); seg_port = 0x78; // Display '7' delay(80); seg_port = 0x00; // Display '8' delay(80); seg_port = 0x10; // Display '9' delay(100); // Default 'H' segment turn ON seg_port = 0xC0; // Display '0' delay(80); seg_port = 0xF9; // Display '1' delay(80); seg_port = 0xA4; // Display '2' delay(80); seg_port = 0xB0; // Display '3' delay(80); seg_port = 0x99; // Display '4' delay(80); seg_port = 0x92; // Display '5' delay(80); seg_port = 0x82; // Display '6' delay(80); seg_port = 0xF8; // Display '7' delay(80); seg_port = 0x80; // Display '8' delay(80); seg_port = 0x90; // Display '9' delay(100); } }